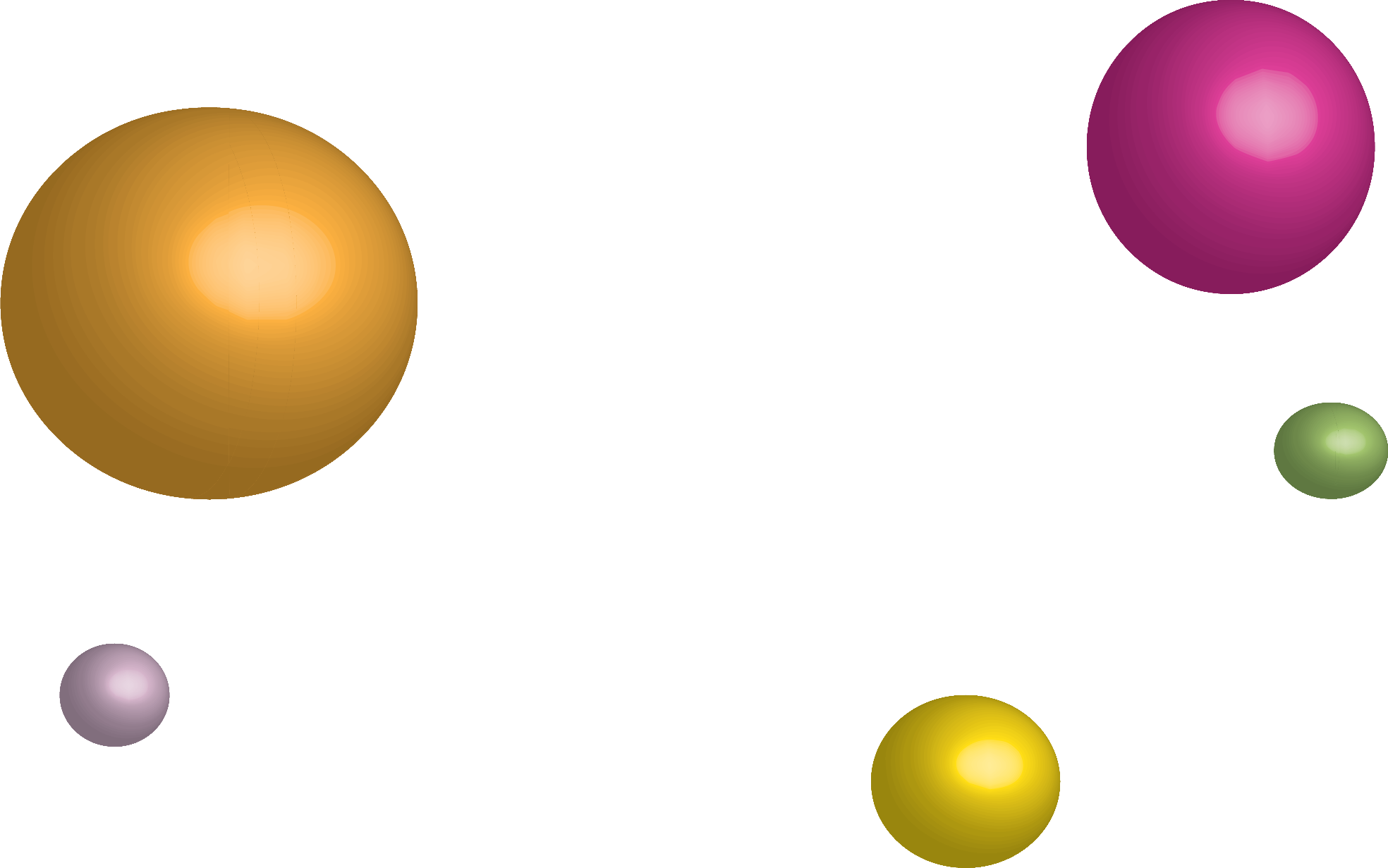
Map as Art
“A map is a tool to both obscure and represent information, to organize space, power and the complexity of life.”
The Ask.
Using Processing software, devise a way to represent the complexity and nuances of your own map in an algorithmic, code-based art piece. Choose a realm of more conceptual interest that allows experimentation & personal interpretation. Can you track something intangible & more elusive than physical space?
The Idea.
My goal for this execution was to find a way to track the changes in the progression of a piece of music by allowing code to bring to life the movements & varying directions that sound consists of. I wanted sound to take a near palpable form in hopes of creating an organic sound map.
The Outcome.
Processing code:
import ddf.minim.analysis.*; // analyzes the audio being played
import ddf.minim.*;
Minim minim; // sound setup
AudioPlayer player;
FFT fft;
float colorAngle = 0.0;
float redX = 233, greenX = 247, blueX = 187;
float redY = 38, greenY = 54, blueY = 11;
float r = redX, g = greenX, b = blueX;
float speed = 0.02;
float[] view1;
float[] view2;
float[] y, x, z;
void setup(){
size(1440, 800, P3D);
minim = new Minim(this);
player = minim.loadFile("phantogram.mp3");
player.play(); // plays audio
fft = new FFT(player.bufferSize(), player.sampleRate()); // buffer size and sample rate store FFT information, sample rate determines the number of data points per second
y = new float[fft.specSize()];
x = new float[fft.specSize()];
z = new float[fft.specSize()];
view1 = new float[fft.specSize()];
view2 = new float[fft.specSize()];
frameRate(100); // refreshes the frame 100 times per second
float smoothingFactor = 0.25;
}
void draw(){
background(r, g, b);
float value1 = sin(colorAngle);
println(value1);
r = map(value1, -1, 1, redX, redY);
g = map(value1, -1, 1, greenX, greenY);
b = map(value1, -1, 1, blueX, blueY);
colorAngle += speed;
lights();
fft.forward(player.mix);
go();
}
void go(){
noStroke();
push();
translate(width/2, height/2); // specifies an amount to displace objects within the display window
for (int i = 0; i < fft.specSize(); i++) {
y[i] = y[i] + fft.getBand(i)/1000; // returns the value of the frequency at position i
x[i] = x[i] + fft.getFreq(i)/500;
z[i] = z[i] + fft.getFreq(i)/700;
view1[i] = view1[i] + fft.getFreq(i)/3000;
fill(fft.getFreq(i)*3, 150, fft.getBand(i)*2);
push();
translate((x[i]*50), (y[i]*50), (z[i]*50));
box(fft.getBand(i)/20+fft.getFreq(i)/15);
pop();
}
pop();
push();
translate(width/2, height/2, 0);
for (int i = 0; i < fft.specSize(); i++){
y[i] = y[i];
x[i] = x[i];
z[i] = z[i];
view2[i] = view2[i]+fft.getFreq(i)/10000;
fill(173, 47-fft.getFreq(i)*2, 76-fft.getBand(i)*2);
push();
translate((x[i]+player.right.get(i)+150), (y[i]+player.right.get(i)+150), (z[i]+player.right.get(i)+150));
box(fft.getBand(i)/10*fft.getFreq(i)/15);
pop();
}
pop();
push();
translate(width/2, height/2, 0);
for (int i = 0; i < fft.specSize(); i++){
y[i] = y[i];
x[i] = x[i];
z[i] = z[i];
view2[i] = view2[i]+fft.getFreq(i)/20000;
fill(232, 232-fft.getFreq(i)*2, 155-fft.getBand(i)*2);
push();
translate((x[i]*200), (y[i]*100), (z[i]*150));
rect(fft.getBand(i)/6, fft.getBand(i)/5, fft.getBand(i)/6, fft.getFreq(i)/6);
pop();
}
pop();
push();
translate(width/5, height/5, 0); // orignally 2
for (int i = 0; i < fft.specSize(); i++){
y[i] = y[i];
x[i] = x[i];
z[i] = z[i];
view2[i] = view2[i]+fft.getFreq(i)/10000;
rotateX(sin(view2[i]/5)); // rotates the shape, positive numbers rotate objects in a clockwise direction
rotateY(cos(view2[i]/5));
rotateZ(sin(view2[i]/5));
fill(217, 249-fft.getFreq(i)*2, 252-fft.getBand(i)*2);
push();
translate((x[i]+250), (y[i]+250), (z[i]+250)); // originally all 250
sphere(fft.getBand(i)/6);
pop();
}
pop();
}
void stop(){
player.close();
minim.stop();
super.stop();
}
// https://code.compartmental.net/minim/minim_class_minim.html
// https://forum.processing.org/one/topic/analyse-sound.html